Menu
You're browsing the documentation for an old version of MoonShine. Consider upgrading your project to MoonShine 2.x.
# Registration
We have already figured out how to register sections of the admin panel to make them appear in the menu
namespace App\Providers; use Illuminate\Support\ServiceProvider;use MoonShine\Menu\MenuItem; use MoonShine\MoonShine; use MoonShine\Resources\MoonShineUserResource;use MoonShine\Resources\MoonShineUserRoleResource; class MoonShineServiceProvider extends ServiceProvider{ //... public function boot() { app(MoonShine::class)->menu([ MenuItem::make('Admins', new MoonShineUserResource()), MenuItem::make('Roles', new MoonShineUserRoleResource()), ]); }}
But to make our interface convenient we can also group menu items
namespace App\Providers; use Illuminate\Support\ServiceProvider;use MoonShine\Menu\MenuItem;use MoonShine\Menu\MenuGroup; use MoonShine\MoonShine;use MoonShine\Resources\MoonShineUserResource;use MoonShine\Resources\MoonShineUserRoleResource; class MoonShineServiceProvider extends ServiceProvider{ //... public function boot() { app(MoonShine::class)->menu([ MenuGroup::make('System', [ MenuItem::make('Admins', new MoonShineUserResource()), MenuItem::make('Roles', new MoonShineUserRoleResource()), ]) ]); }}
You just need to add the resources as a second parameter to the MoonShine\Menu\MenuGroup
class.
And the first parameter is the name of the group!
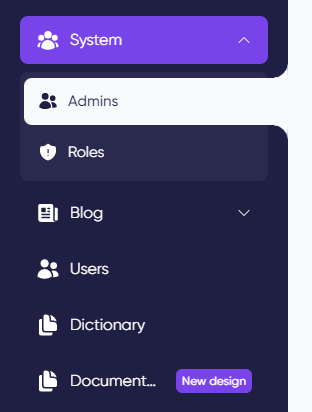
# Condition-based display
Displays menu based on the condition
//...app(MoonShine::class)->menu([ MenuGroup::make('System', [ MenuItem::make('Admins', new MoonShineUserResource()), MenuItem::make('Roles', new MoonShineUserRoleResource()), ])->canSee(function(Request $request) { return $request->user('moonshine')?->id === 1; }) ]);//...
# External link
Adding a custom link
//...app(MoonShine::class)->menu([ MenuItem::make('Документация Laravel', 'https://laravel.com') ]);//...
Links can be passed through a function
//...app(MoonShine::class)->menu([ MenuItem::make('Admins', function () { return (new MoonShineUserResource())->route('index'); }), MenuItem::make('Home', fn() => route('home')) ]);//...
# Icon
You can also change the icon of the menu item
//...app(MoonShine::class)->menu([ MenuGroup::make('Система', [ MenuItem::make('Admins', new MoonShineUserResource())->icon('heroicons.hashtag'), MenuItem::make('Roles', new MoonShineUserRoleResource())->icon('heroicons.hashtag'), ])->icon('app') // or MenuGroup::make('Blog', [ MenuItem::make('Comments', new CommentResource(), 'heroicons.chat-bubble-left') ], 'heroicons.newspaper') ]);//...
For more information, see Icons
# Tag
You can add a counter to the menu item
//...app(MoonShine::class)->menu([ MenuItem::make('Comments', new CommentResource()) ->badge(fn() => Comment::query()->count()), ]);//...
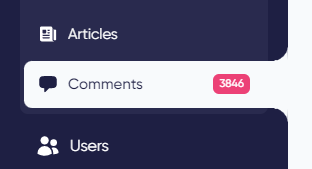
# Translation
To translate menu items, you need to add the translation key as the name
and add the translatable()
method
//...app(MoonShine::class)->menu([ MenuItem::make('menu.Comments', new CommentResource()) ->translatable() // or MenuItem::make('Comments', new CommentResource()) ->translatable('menu') ]);//...
// lang/ru/menu.php return [ 'Comments' => 'Комментарии',];
To translate menu labels, you can use Laravel's translation tools
//...app(MoonShine::class)->menu([ MenuItem::make('Comments', new CommentResource()) ->badge(fn() => __('menu.badge.new')) ]);//...
# Divider
Menu items can be visually separated using MenuDivider
use MoonShine\Menu\MenuDivider; //...app(MoonShine::class)->menu([ MenuItem::make('Categories', new CategoryResource()), MenuDivider::make(), MenuItem::make('Articles', new ArticleResource()),]);//...
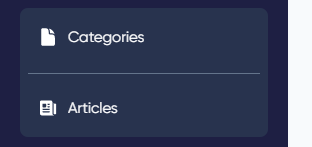
You can use text as a separator, for this you need to pass it to the make()
method
use MoonShine\Menu\MenuDivider; //...app(MoonShine::class)->menu([ MenuItem::make('Categories', new CategoryResource()), MenuDivider::make('Divider'), MenuItem::make('Articles', new ArticleResource()),]);//...
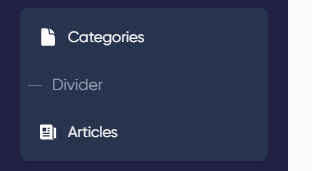