WYSIWYG
You're browsing the documentation for an old version of MoonShine. Consider upgrading your project to MoonShine 2.x.
# TinyMce
use MoonShine\Fields\TinyMce; //...public function fields(): array{ return [ TinyMce::make('Description', 'description'), // More advanced settings TinyMce::make('Text') // Override the plugin set ->plugins('anchor') // Adding plugins to the base set ->addPlugins('code codesample') // Override the set toolbar ->toolbar('undo redo | blocks fontfamily fontsize') // Adding a toolbar to the base set ->addToolbar('code codesample') // To change the author name for a plugin tinycomments ->commentAuthor('Danil Shutsky') // Tags ->mergeTags([ ['value' => 'tag', 'title' => 'Title'] ]) // Override the current locale ->locale('en'), ];}//...
Translation files are placed in the public/vendor/moonshine/libs/tinymce/langs
directory
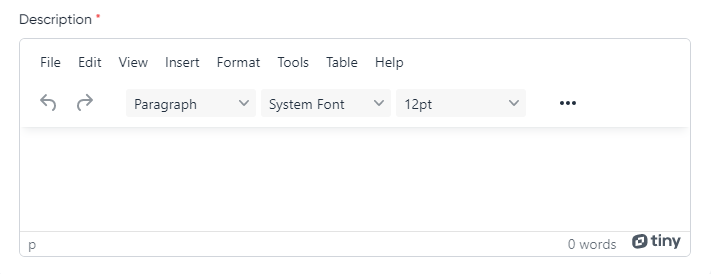
Sign up at
Tiny.Cloud
and get a token. Then add it to config/moonshine.php
config
//...'tinymce' => [ 'token' => 'YOUR_TOKEN' ]//...
# Laravel File manager
Laravel FileManagerIf you want to use the file manager in tinymce, you need to install the Laravel FileManager package
1 Installing
composer require unisharp/laravel-filemanager php artisan vendor:publish --tag=lfm_configphp artisan vendor:publish --tag=lfm_public
Be sure to set the 'use_package_routes' flag in the lfm config to false, otherwise the route caching will cause an error
return [ // ... 'use_package_routes' => false, // ...];
2 Add routes to the app/Providers/RouteServiceProvider.php
// ..Route::middleware('web')->group(base_path('routes/web.php')); Route::group(['prefix' => 'laravel-filemanager', 'middleware' => ['moonshine', 'auth.moonshine']], function () { UniSharp\LaravelFilemanager\Lfm::routes();}); // ..
The file manager route must be in the moonshine
middleware group, not in the web!
To allow access only to users authorized in the admin panel
middleware auth.moonshine
must be used
3 Add the prefix to config/moonshine.php
//...'tinymce' => [ 'file_manager' => 'laravel-filemanager', // ...]//...
# Trix
This field belongs to a separate package, complete the installation before use
composer require moonshine/trix
use MoonShine\Fields\Trix; //...public function fields(): array{ return [ Trix::make('Description', 'description'), ];}//...
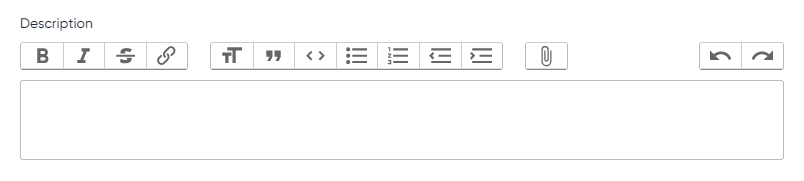
# CKEditor
The field is placed in a separate package, before use it is necessary to perform the installation
composer require moonshine/ckeditor
use MoonShine\CKEditor\Fields\CKEditor; //...public function fields(): array{ return [ CKEditor::make('Description', 'description'), ];}//...
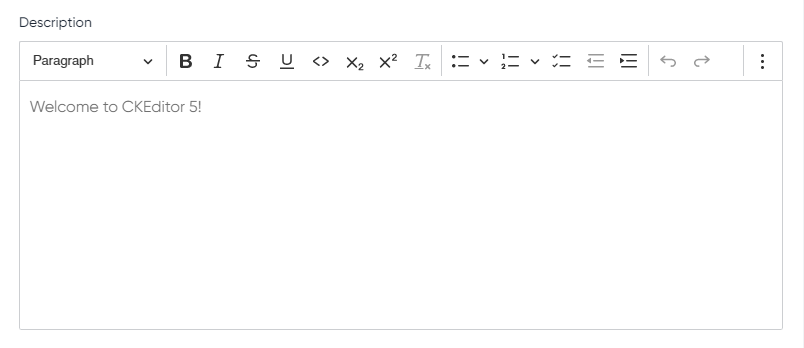
# Quill
The field is placed in a separate package, before use it is necessary to perform the installation
composer require moonshine/quill
use MoonShine\Quill\Fields\Quill; //...public function fields(): array{ return [ Quill::make('Description', 'description'), ];}//...
