Item actions
You're browsing the documentation for an old version of MoonShine. Consider upgrading your project to MoonShine 2.x.
# Registration
By default, the table in the MoonShine panel contains only 2 actions for items - edit and delete. But you can also add your own custom actions
namespace MoonShine\Resources; use MoonShine\Models\MoonshineUser;use MoonShine\ItemActions\ItemAction; class PostResource extends Resource{ public static string $model = App\Models\Post::class; public static string $title = 'Articles'; //... public function itemActions(): array { return [ ItemAction::make('Deactivating', function (Model $item) { $item->update(['active' => false]); }, 'Deactivated')->icon('app') ]; } //...}
The first argument is the name of the action. The second argument is the callback with the current item parameter. The third argument - the message that will be displayed after the action is executed
# Display condition
Display action by condition
...public function itemActions(): array{ return [ ItemAction::make('Restore', function (Model $item) { $item->restore(); }, 'Retrieved') ->canSee(fn(Model $item) => $item->trashed()) ];}...
# Display method
To display actions as a dropdown list, you can use the showInDropdown
method
use MoonShine\ItemActions\ItemAction; //...public function itemActions(): array{ return [ ItemAction::make('Deactivation', function (Model $item) { $item->update(['active' => false]); }, 'Deactivated') ->showInDropdown() ];}//...
This display method is used by default
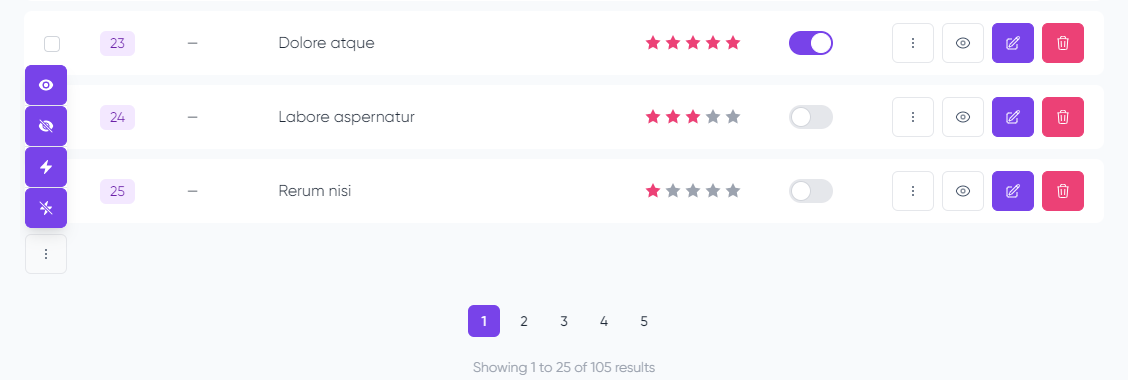
To display actions as a horizontal list, you can use the showInLine
method
use MoonShine\ItemActions\ItemAction; //...public function itemActions(): array{ return [ ItemAction::make('Deactivation', function (Model $item) { $item->update(['active' => false]); }, 'Deactivated') ->showInLine() ];}//...
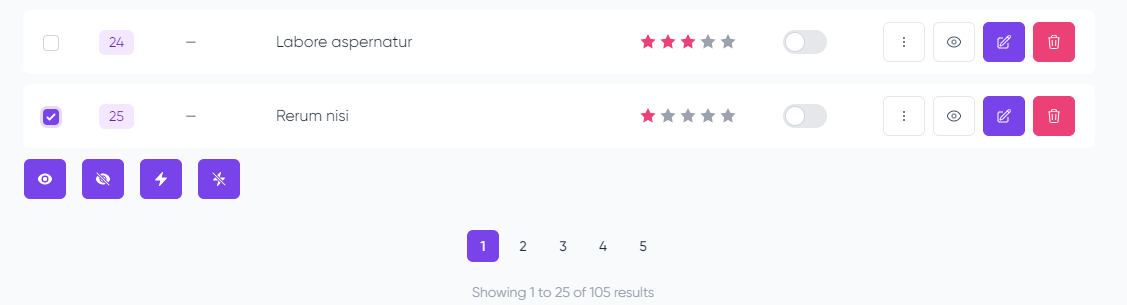
# Confirm the action
To confirm the action, you must use the withConfirm
method
use MoonShine\ItemActions\ItemAction; //...public function itemActions(): array{ return [ ItemAction::make('Deactivation', function (Model $item) { $item->update(['active' => false]); }, 'Deactivated') ->withConfirm() ];}//...
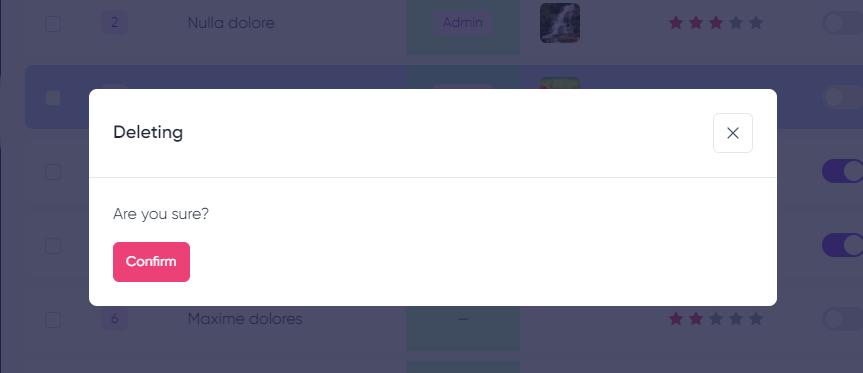
The withConfirm()
method can be passed the title and text for the modal window, as well as the title
for confirmation button
use MoonShine\ItemActions\ItemAction; //...public function itemActions(): array{ return [ ItemAction::make('Deactivation', function (Model $item) { $item->update(['active' => false]); }, 'Deactivated') ->withConfirm('Title', 'Modal content', 'Accept') ];}//...
The errorMessage()
method allows you to set the text of the error message
use MoonShine\ItemActions\ItemAction; //...public function itemActions(): array{ return [ ItemAction::make('Deactivation', function (Model $item) { $item->update(['active' => false]); }, 'Deactivated') ->withConfirm('Title', 'Modal content', 'Accept') ->errorMessage('Fail') ];}//...