Form actions
You're browsing the documentation for an old version of MoonShine. Consider upgrading your project to MoonShine 2.x.
-
Sections
By default, forms in the MoonShine panel contain only 1 action - saving. But you can add your own custom actions
namespace MoonShine\Resources; use MoonShine\Models\MoonshineUser;use MoonShine\FormActions\FormAction; class PostResource extends Resource{ public static string $model = App\Models\Post::class; public static string $title = 'Articles'; //... public function formActions(): array { return [ FormAction::make('Delete', function (Model $item) { $item->delete(); }, 'Removed')->icon('delete') ]; } //...}
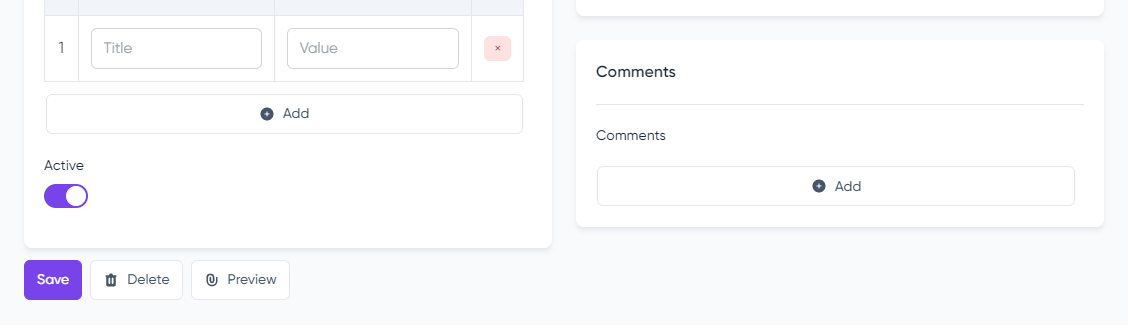
# Display method
To display actions as a dropdown list, you can use the showInDropdown
method
use MoonShine\FormActions\FormAction; //...public function formActions(): array{ return [ FormAction::make('Deactivation', function (Model $item) { $item->update(['active' => false]); }, 'Deactivated') ->showInDropdown() ];}//...
This display method is used by default
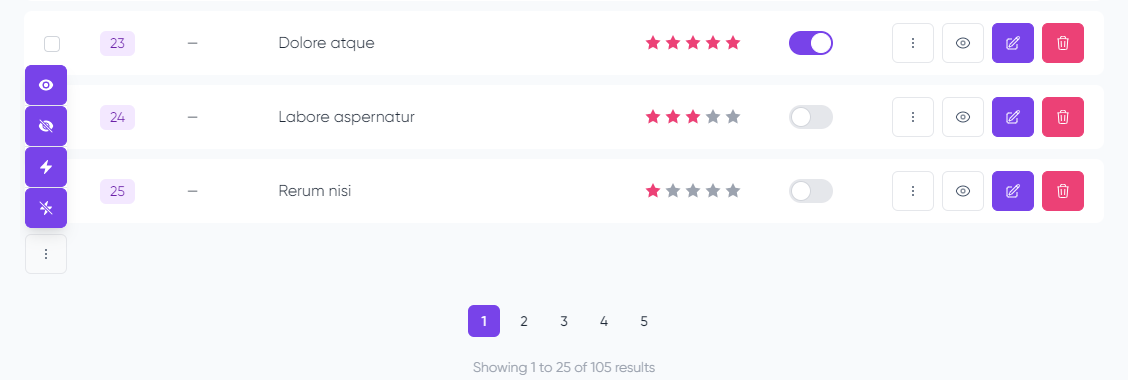
To display actions as a horizontal list, you can use the showInLine
method
use MoonShine\FormActions\FormAction; //...public function formActions(): array{ return [ FormAction::make('Deactivation', function (Model $item) { $item->update(['active' => false]); }, 'Deactivated') ->showInLine() ];}//...
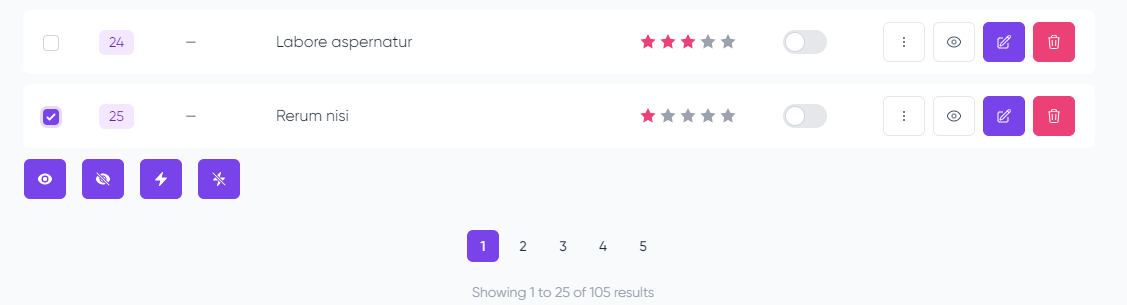
# Confirm the action
To confirm the action, you must use the withConfirm
method
use MoonShine\FormActions\FormAction; //...public function formActions(): array{ return [ FormAction::make('Deactivation', function (Model $item) { $item->update(['active' => false]); }, 'Deactivated') ->withConfirm() ];}//...
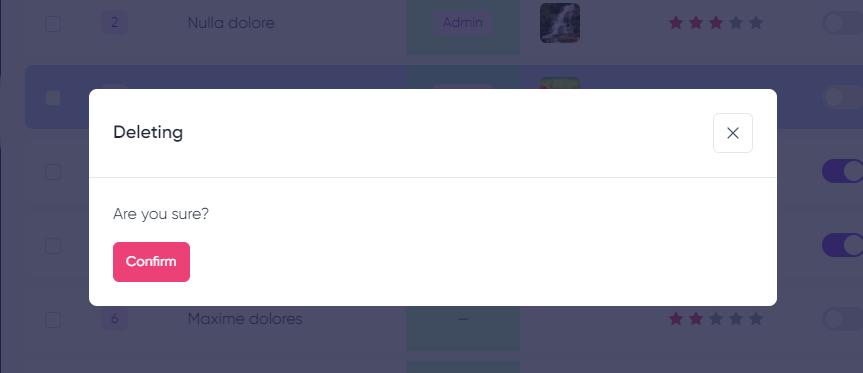
The withConfirm()
method can be passed the title and text for the modal window, as well as the title
for confirmation button
use MoonShine\FormActions\FormAction; //...public function formActions(): array{ return [ FormAction::make('Deactivation', function (Model $item) { $item->update(['active' => false]); }, 'Deactivated') ->withConfirm('Title', 'Modal content', 'Accept') ];}//...
The errorMessage()
method allows you to set the text of the error message
use MoonShine\FormActions\FormAction; //...public function formActions(): array{ return [ FormAction::make('Deactivation', function (Model $item) { $item->update(['active' => false]); }, 'Deactivated') ->withConfirm('Title', 'Modal content', 'Accept') ->errorMessage('Fail') ];}//...