Basics
You're browsing the documentation for an old version of MoonShine. Consider upgrading your project to MoonShine 2.x.
-
Sections
# Adding a filter
Extends
Fields
* has the same features
Filters are shown on the main page of the resource to filter data. They implement from the corresponding fields, so all methods of these fields are available here as well.
Filters work in exactly the same way as fields, except that they are declared in the
filters
resource method
use MoonShine\Filters\BelongsToFilter;use MoonShine\Filters\BelongsToManyFilter;use MoonShine\Filters\DateRangeFilter;use MoonShine\Filters\SlideFilter;use MoonShine\Filters\SwitchBooleanFilter;use MoonShine\Filters\TextFilter; //... public function filters(): array{ return [ TextFilter::make('Title'), BelongsToFilter::make('Author', resource: 'name') ->nullable() ->canSee(fn() => auth('moonshine')->user()->moonshine_user_role_id === 1), TextFilter::make('Slug'), BelongsToManyFilter::make('Categories') ->select(), DateRangeFilter::make('Created at'), SlideFilter::make('Age') ->fromField('age_from') ->toField('age_to') ->min(0) ->max(60), SwitchBooleanFilter::make('Active') ];} //...
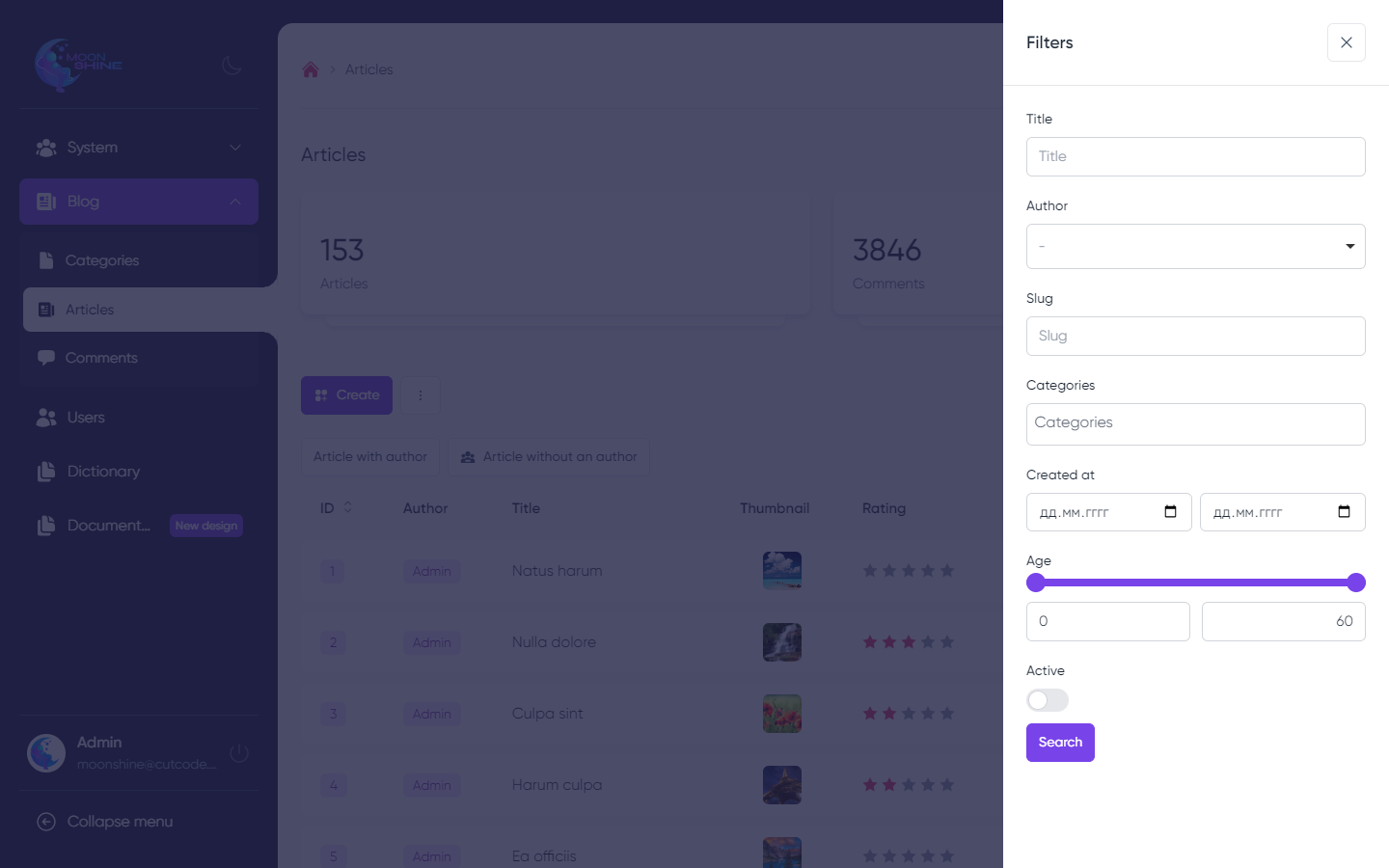
# Custom query
Using the customQuery
method, you can create a custom query for the filter
use MoonShine\Filters\DateRangeFilter;use MoonShine\Filters\TextFilter; //... public function filters(): array{ return [ TextFilter::make('Title') ->customQuery(fn(Builder $query, $value) => $query->where('title', 'LIKE', "%${value}%")), DateRangeFilter::make('Created at') ->customQuery(function (Builder $query, $values) { return $query ->when($values['from'] ?? null, function ($query, $fromDate) { $query->whereDate('created_at', '>=', Carbon::parse($fromDate)); }) ->when($values['to'] ?? null, function ($query, $toDate) { $query->whereDate('created_at', '<=', Carbon::parse($toDate)); }); }), ];} //...