BelongsToMany
You're browsing the documentation for an old version of MoonShine. Consider upgrading your project to MoonShine 2.x.
-
Sections
# Basics
Field for relationships in Laravel, BelongsToMany
type.
Displayed as a group of checkboxes, you can also transform into select multiple.
use MoonShine\Fields\BelongsToMany; //...public function fields(): array{ return [ BelongsToMany::make('Categories', 'categories', 'name') ];}//...
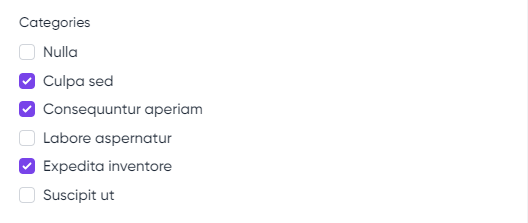
# Pivot
To implement pivot fields, use the fields()
method.
use MoonShine\Fields\BelongsToMany; //...public function fields(): array{ return [ BelongsToMany::make('Contacts', 'contacts', 'name') ->fields([ Text::make('Contact', 'text'), ]) ];}//...
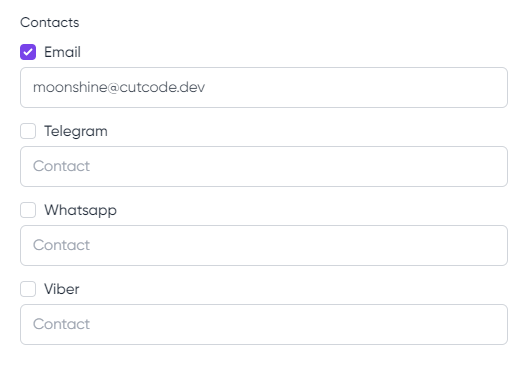
# Async search
Async search for values is provided by the asyncSearch()
method.
use MoonShine\Fields\BelongsToMany; //...public function fields(): array{ return [ BelongsToMany::make('Contacts') ->asyncSearch()->fields([Text::make('Contact', 'text')]) ];}//...
The search will be performed on the resource relation field titleField
. The default is titleField=id
.
You can pass parameters to the asyncSearch()
method:
title
- search fieldcount
- amount of items in outputasyncSearchQuery()
- callback function for filtering valuesasyncSearchValueCallback()
- callback function for output customization.
use Illuminate\Contracts\Database\Eloquent\Builder; use MoonShine\Fields\BelongsToMany; //...public function fields(): array{ return [ BelongsToMany::make('Contacts')->asyncSearch( 'title', 10, asyncSearchQuery: function (Builder $query) { return $query->where('id', '!=', 2); }, asyncSearchValueCallback: function ($contact) { return $contact->id . ' | ' . $contact->title; } )->fields([Text::make('Contact', 'text')]) ];}//...
When building a query in asyncSearchQuery()
, you can use the current form values.
To do this, you need to pass Request
to the callback functions.
use Illuminate\Contracts\Database\Eloquent\Builder; use Illuminate\Http\Request; use MoonShine\Fields\BelongsToMany;use MoonShine\Fields\Select; //...public function fields(): array{ return [ Select::make('Country', 'country_id'), BelongsToMany::make('City')->asyncSearch( 'title', asyncSearchQuery: function (Builder $query, Request $request): Builder { return $query->where('country_id', $request->get('country_id')); } ) ];}//...
Relations that use async search in their fields are recommended to be used in ResourceMode
.
Requests must be customized via the asyncSearch()
method,
don't use valuesQuery()
!
# Select
To transform the display into select, use the select()
method.
use MoonShine\Fields\BelongsToMany; //...public function fields(): array{ return [ BelongsToMany::make('Categories', 'categories', 'name') ->select() ];}//...
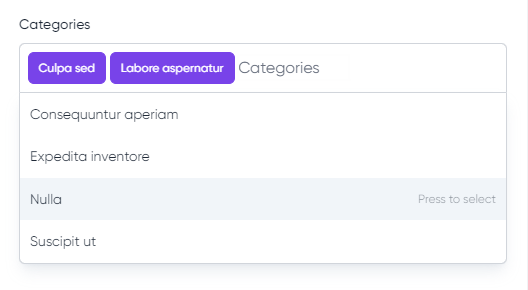
# Values query
To filter values, use thevaluesQuery
method
use Illuminate\Contracts\Database\Eloquent\Builder; use MoonShine\Fields\BelongsToMany; //...public function fields(): array{ return [ BelongsToMany::make('Categories', 'categories', 'name') ->valuesQuery(fn(Builder $query) => $query->where('active', true)) ];}//...
# Tree
Sometimes it makes sense to display checkboxes with a hierarchy, example for categories that have nesting,
there is a tree()
method for this.
use MoonShine\Fields\BelongsToMany; //...public function fields(): array{ return [ BelongsToMany::make('Categories', 'categories', 'name') ->tree('parent_id') // Contact field // ];}//...
# onlyCount
If you want to display only the number of selected values on the index page,
then you should use the onlyCount()
method.
use MoonShine\Fields\BelongsToMany; //...public function fields(): array{ return [ BelongsToMany::make('Categories', 'categories', 'name') ->onlyCount() ];}//...
# inLine
By default, the index page will display the field as a table,
but if you want to display it in a line, you can use the inLine()
method.
Optional parameters can be passed to the method:
separator
- separator between elementsbadge
- display elements as badge
use MoonShine\Fields\BelongsToMany; //...public function fields(): array{ return [ BelongsToMany::make('Categories', 'categories', 'name') ->inLine(separator: ' ', badge: true) ];}//...
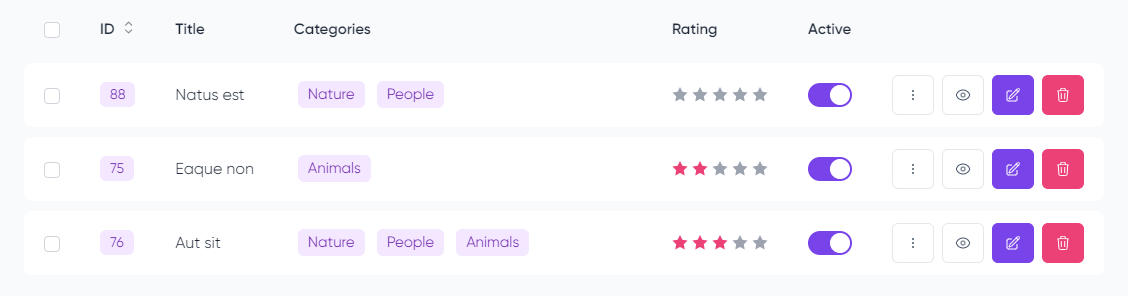