NoInput
You're browsing the documentation for an old version of MoonShine. Consider upgrading your project to MoonShine 2.x.
-
Sections
The field is not for data input/amendment!
# Basic application
With this default field, you can display text data from any field in the model, or generate text based on the model.
//...use MoonShine\Fields\NoInput; public function fields(): array{ return [ NoInput::make('No input field', 'no_input', static fn() => fake()->realText()), ];} //...
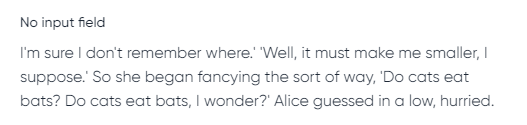
# Badge
Displays a label, could be used for order status, as an example! We use the badge method with a color parameter, which can be either a string or a closure with the current element in the parameter
//...use MoonShine\Fields\NoInput; public function fields(): array{ return [ NoInput::make('Status')->badge(fn($item) => $item->status_id === 1 ? 'green' : 'gray'), ];} //...
# Boolean
Display a label (green or red) for boolean values. Using the hideTrue and hideFalse options you can hide the label for values input
//...use MoonShine\Fields\NoInput; public function fields(): array{ return [ NoInput::make('Active')->boolean(hideTrue: false, hideFalse: false), ];} //...
# Link
Displays a link. We can show the value and specify the link using the parameter (string or closure with the current element)
//...use MoonShine\Fields\NoInput; public function fields(): array{ return [ NoInput::make('Link')->link('https://cutcode.dev', blank: false), NoInput::make('Link')->link(fn($item) => $item->link, blank: true), ];} //...
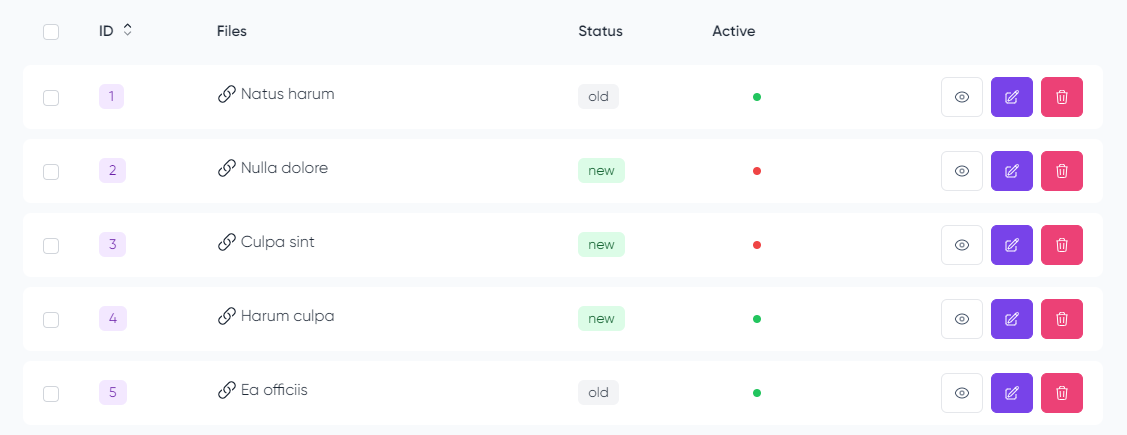