File
You're browsing the documentation for an old version of MoonShine. Consider upgrading your project to MoonShine 2.x.
Before using the file field, make sure that a symbolic link is set to the storage directory
php artisan storage:link
use MoonShine\Fields\File; //...public function fields(): array{ return [ //... File::make('File', 'file') ->dir('/') // The directory where the files will be stored in storage (by default /) ->disk('public') // Filesystems disk ->allowedExtensions(['jpg', 'gif', 'png']) // Allowable extensions //... ];}//...
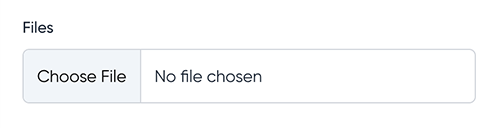
To correctly generate the file URL, you must define the environment variable APP_URL
in this way,
to match your app's URL.
When using the local
driver, the return value of url
is not URL encoded.
For this reason, we recommend always storing your files using names that will create valid URLs.
# Multiple
The multiple()
method is used to upload multiple files
use MoonShine\Fields\File; //...public function fields(): array{ return [ //... File::make('File', 'file') ->multiple(), //... ];}//...
The field in the database must be of text or json type.
You also need to add cast for eloquent model - json or array or collection.
# Removing files
To be able to delete files, you must use the removable()
method
use MoonShine\Fields\File; //...public function fields(): array{ return [ //... File::make('File', 'file') ->removable(), //... ];}//...
The disableDeleteFiles()
method will allow you to delete only the record in the database,
but not delete the file itself
use MoonShine\Fields\File; //...public function fields(): array{ return [ //... File::make('File', 'file') ->removable() ->disableDeleteFiles(), //... ];}//...
The enableDeleteDir()
method deletes the directory specified in the dir()
method if it is empty
use MoonShine\Fields\File; //...public function fields(): array{ return [ //... File::make('File', 'file') ->dir('/images/') ->removable() ->enableDeleteDir(), //... ];}//...
# Disabling download
If you want to protect the file from download, you must use the disableDownload()
method
use MoonShine\Fields\File; //...public function fields(): array{ return [ //... File::make('File', 'file') ->disableDownload(), //... ];}//...
# Original filename
If you want to keep the original filename received from the client, use the keepOriginalFileName()
method
use MoonShine\Fields\File; //...public function fields(): array{ return [ //... File::make('File', 'file') ->keepOriginalFileName(), //... ];}//...
# Custom file name
If you need to save a custom file name, use the method customName('file_name'))
use MoonShine\Fields\File;use Illuminate\Http\UploadedFile;use Illuminate\Support\Str; //...public function fields(): array{ return [ //... File::make('File', 'file') ->customName(fn(UploadedFile $file) => Str::random(10) . '.' . $file->extension()), //... ];}//...