Json
You're browsing the documentation for an old version of MoonShine. Consider upgrading your project to MoonShine 2.x.
-
Sections
In the database, the field must be of type text or json. Also cast eloquent array or json or collection models.
# Key/Value
The easiest way is to use the keyValue method, in which case the database will have a simple json [{key: value}]
use MoonShine\Fields\Json; //...public function fields(): array{ return [ Json::make('Product Options', 'options') ->keyValue('Characteristic', 'Value') // First argument Key label, second argument Value label ];}//...
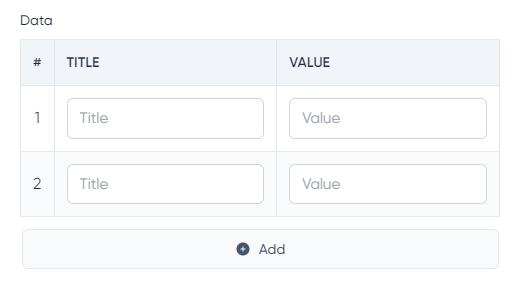
# With a set of fields
For more advanced use, use the fields method and pass the required set of fields in a similar way to how the resource works
use MoonShine\Fields\Json; //...public function fields(): array{ return [ Json::make('Product Options', 'options') ->fields([ Text::make('Title', 'title'), Text::make('Value', 'value') ]) ];}//...
json [{title: 'value', value: 'value'}]
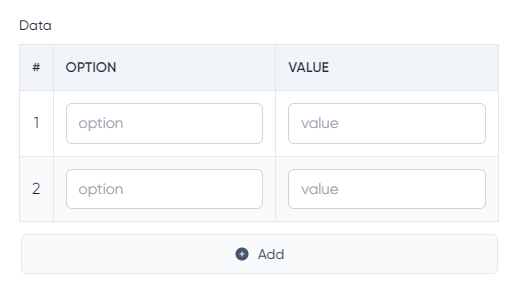
# Deleting
Json::make('Product Options', 'options') ->keyValue('Characteristics', 'Value') ->removable()
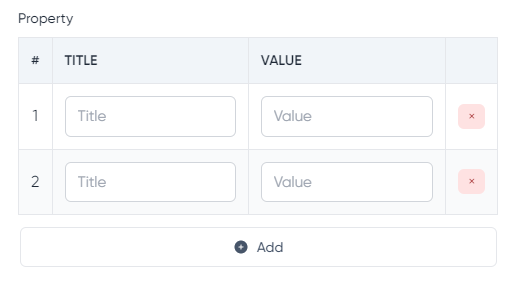
# Value only
Sometimes you only need to store the values in the database
for this you can use the onlyValue()
method.
Json::make('Product Options', 'options') ->onlyValue()