Actions
You're browsing the documentation for an old version of MoonShine. Consider upgrading your project to MoonShine 2.x.
-
Sections
# Basics
Often it is necessary to do something with the list of the section and "Actions" serve for this purpose. At the moment, there is only one Action class in MoonShine, which is responsible for exporting data, but actions could be extended and you can easily write your own.
You can simply return all the necessary actions in the actions
method which returns an array.
And we will talk about actions structure in the
"Actions"
section.
If the method is absent or returns an empty array, then the actions will not be displayed
namespace MoonShine\Resources; use MoonShine\Models\MoonshineUser;use MoonShine\Actions\ExportAction; class PostResource extends Resource{ public static string $model = App\Models\Post::class; public static string $title = 'Articles'; //... public function actions(): array { return [ ExportAction::make('Export') ]; } //...}

# Display method
To display actions as a dropdown list, you can use the showInDropdown
method
use MoonShine\Actions\ExportAction; //...public function actions(): array{ return [ ExportAction::make('Export') ->showInDropdown() ];}//...
This display method is used by default
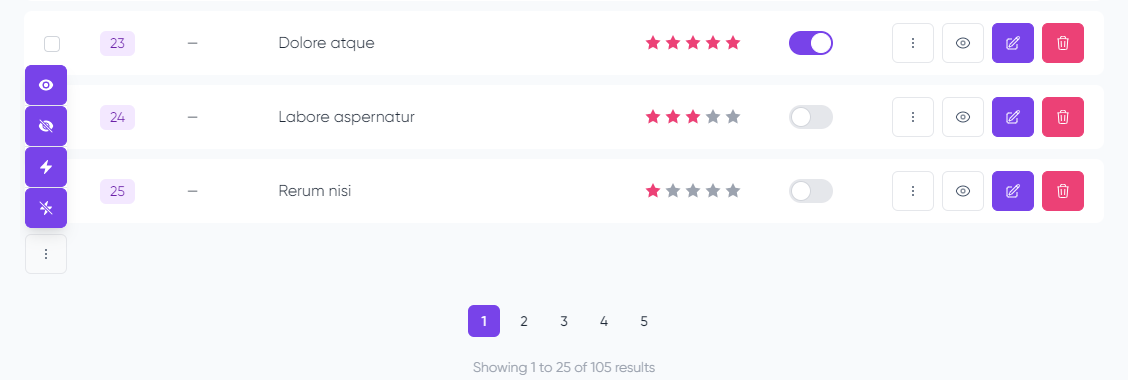
To display actions as a horizontal list, you can use the showInLine
method
use MoonShine\Actions\ExportAction; //...public function actions(): array{ return [ ExportAction::make('Export') ->showInLine() ];}//...
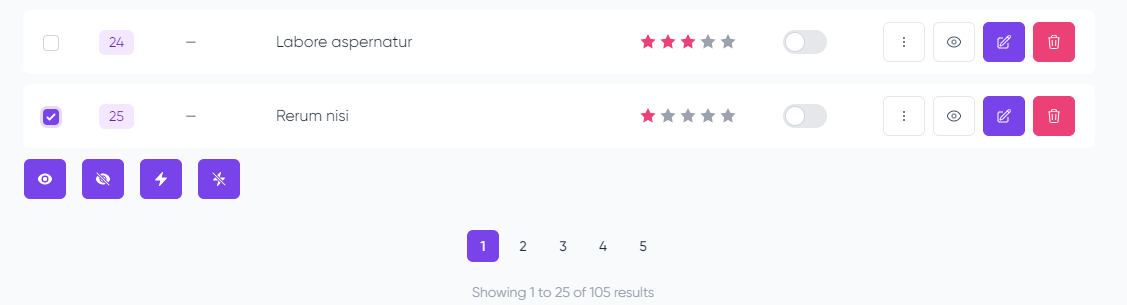